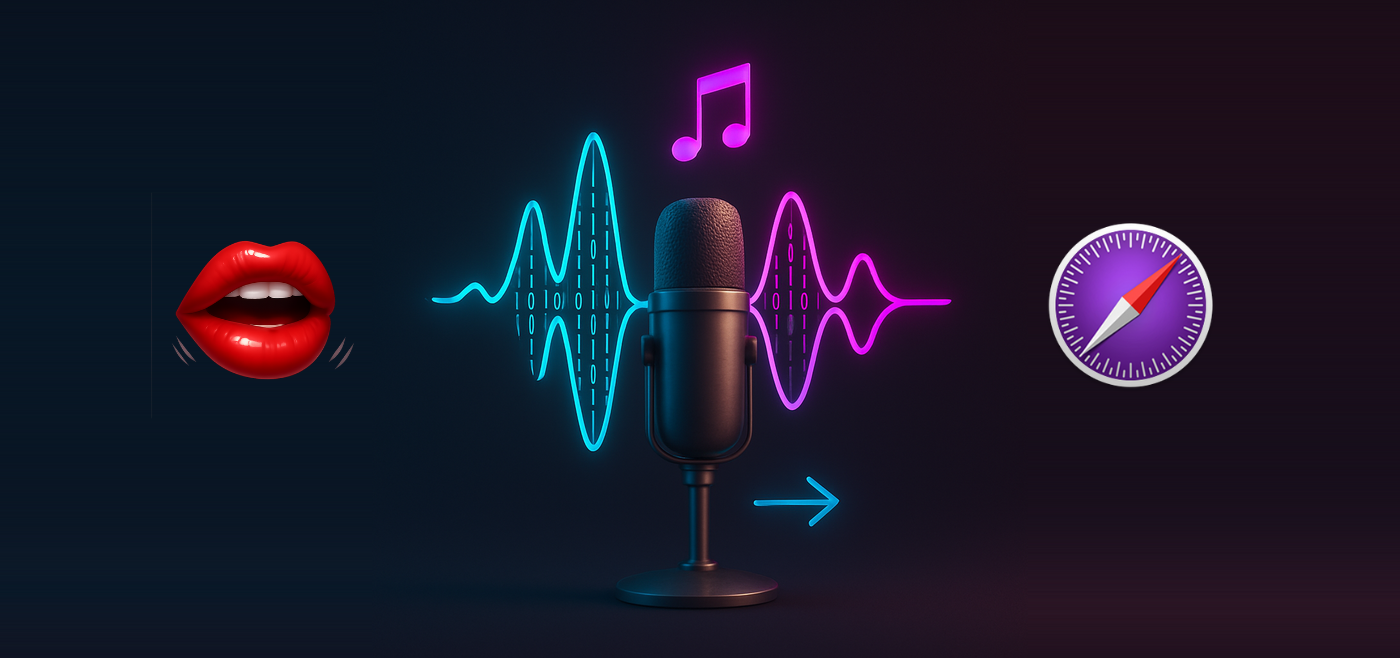
Record Lossless Audio in Safari Technology Preview With New ALAC and PCM Codec Support
In Safari Technology Preview Release 214, Apple introduced support for ALAC (Apple Lossless Audio Codec) and PCM (Pulse Code Modulation) audio codecs in the MediaRecorder API. This enhancement lets developers record high-quality, lossless audio directly within the Safari browser. The release version of Safari supports AAC.
Understanding ALAC and PCM lets developers
- ALAC (Apple Lossless Audio Codec): Developed by Apple, ALAC is a lossless audio compression format that preserves the original audio data without any quality loss. Depending on the audio content, it achieves approximately 40-60% compression of the original file size. ALAC files are typically stored with the
.m4a
or.caf
extensions. - PCM (Pulse Code Modulation): PCM is a method used to digitally represent analog signals. It is considered a lossless format because it captures audio data without compression, resulting in larger file sizes. PCM files are typically stored with the
.wav
or.aiff
extensions.
Generating ALAC and PCM Recordings in Safari
Developers can specify the desired audio format using the mimeType
property. To record audio in ALAC or PCM, set the mimeType
accordingly:
navigator.mediaDevices.getUserMedia({ audio: true })
.then(stream => {
const mimeType = 'audio/mp4; codecs=alac'; // Use 'audio/mp4; codecs=pcm' for PCM
if (!MediaRecorder.isTypeSupported(mimeType)) {
console.error(`MIME type ${mimeType} is not supported.`);
return;
}
const mediaRecorder = new MediaRecorder(stream, { mimeType });
const chunks = [];
mediaRecorder.ondataavailable = event => {
if (event.data.size > 0) {
chunks.push(event.data);
}
};
mediaRecorder.onstop = () => {
const blob = new Blob(chunks, { type: mimeType });
const url = URL.createObjectURL(blob);
const a = document.createElement('a');
a.style.display = 'none';
a.href = url;
a.download = mimeType.includes('alac') ? 'recording.m4a' : 'recording.wav';
document.body.appendChild(a);
a.click(); // Download the recording
window.URL.revokeObjectURL(url);
};
mediaRecorder.start();
setTimeout(() => mediaRecorder.stop(), 5000); // Record for 5 seconds
})
.catch(error => {
console.error('Error accessing media devices.', error);
});
Key Points:
- MIME Type Specification: The
mimeType
is set toaudio/mp4; codecs=alac
for ALAC recordings andaudio/mp4; codecs=pcm
for PCM recordings. - File Extension: The downloaded file uses the
.m4a
extension for ALAC and.wav
for PCM. - Support Verification: Before initializing the MediaRecorder, the code checks if the specified MIME type is supported using
MediaRecorder.isTypeSupported()
.
Live Demo
Our MediaRecorder API based audio recording demo allows you to record audio using the ALAC or PCM audio codecs (also with Opus and AAC).
Pros and Cons of Using ALAC and PCM
Pros:
- Lossless Quality: Both formats preserve the original audio quality, making them ideal for high-fidelity sound applications.
- Compatibility: ALAC is well-supported across Apple devices and software, while PCM is a standard format compatible with various platforms and devices.
Cons:
- File Size: Lossless formats result in larger file sizes compared to lossy formats like AAC or MP3, which may impact storage and bandwidth usage.
- Processing Power: Decoding ALAC files can be more CPU-intensive compared to other lossless formats.
- Support:
M4A
is not always compatible with Windows apps and devices because it is an Apple design.
Browser Support for ALAC and PCM Codecs
The support for ALAC and PCM codecs in the MediaRecorder API varies across browsers. The table below summarizes the compatibility:
Browser | ALAC Support | PCM Support |
---|---|---|
Chrome | Not Supported (135) | Supported (135+) |
Firefox | Not Supported (137) | Not Supported (137) |
Safari | Supported (Safari Technology Preview 214 and later) | Supported (Safari Technology Preview 214 and later) |
Edge | Not Supported (135) | Supported (135+) |
Note: Browser support is subject to change. Refer to the latest browser documentation or use MediaRecorder.isTypeSupported()
to verify support.
Considerations
ALAC offers a balance between compression and quality, making it suitable for applications where storage space is a concern. However, it is mostly not supported outside of the Apple ecosystem. PCM provides uncompressed audio, ensuring the highest quality but at the cost of larger file sizes.