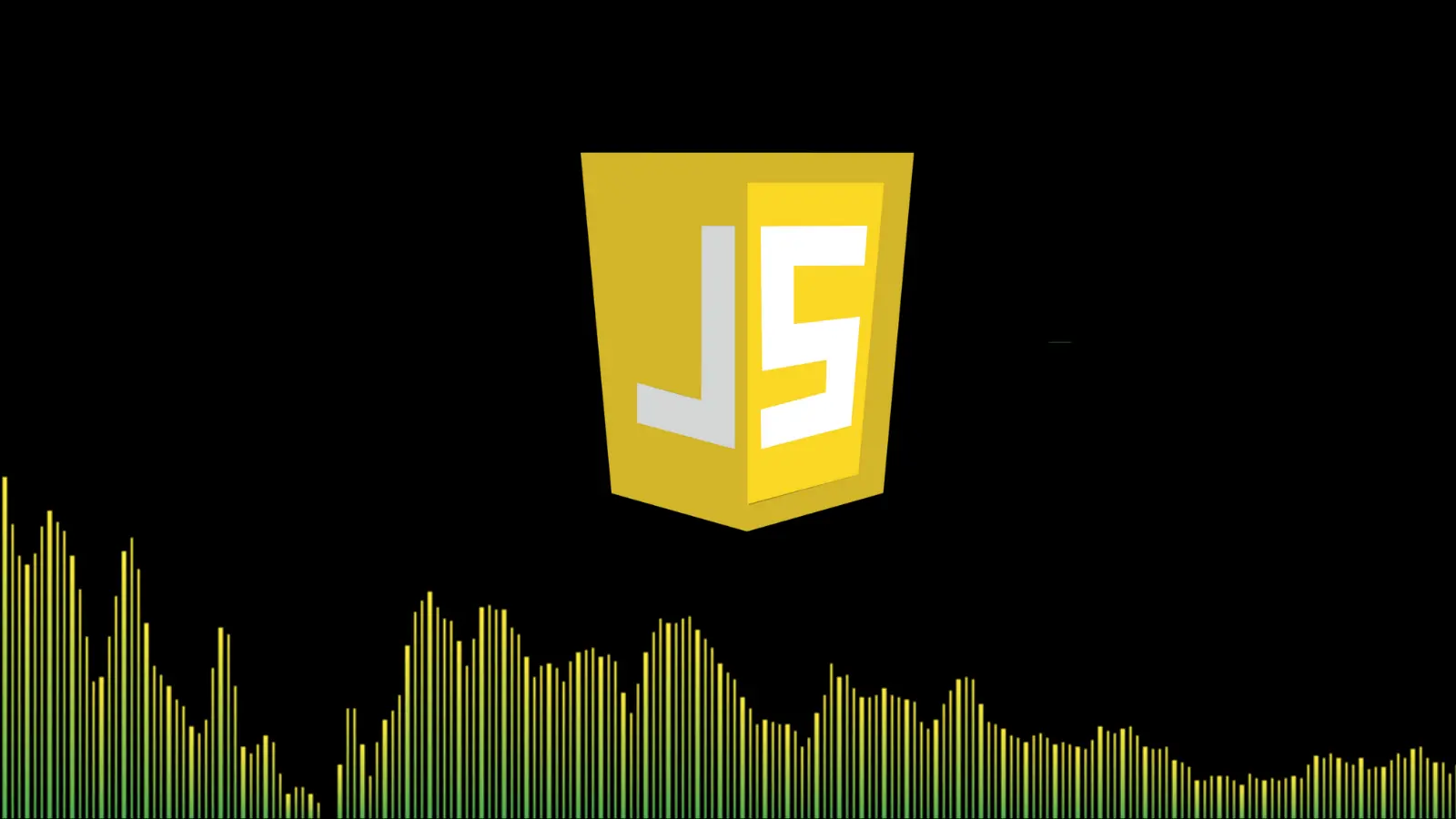
Understanding Audio Frequency Analysis in JavaScript: A Guide to Using AnalyserNode and getByteFrequencyData
One of the most powerful tools available for working with audio in JavaScript is the ability to analyze audio frequency data in real-time. This allows developers to create visualizers, detect specific frequencies, or build advanced application audio meters. In this guide, we’ll walk through the mechanics of using AnalyserNode and its method getByteFrequencyData() to extract meaningful audio data, breaking it down step-by-step for clarity.
The Basics: Capturing and Analyzing Audio Data
We rely on the AnalyserNode interface to analyze audio data, which processes an audio stream and outputs frequency-domain or time-domain data. Here’s how it works:
- Audio Input: We use an AudioWorkletNode in order to get the required audio data for processing.
- Frequency Analysis: We leverage the AnalyserNode’s getByteFrequencyData() method to break down the audio into frequency bins.
Key Concepts in Frequency Analysis
AnalyserNode: fftSize Property
The fftSize determines how detailed the frequency analysis is:
- Definition: The size of the Fast Fourier Transform (FFT) used by the AnalyserNode.
- Values: Must be a power of 2 (e.g., 32, 64, 128… up to 32768).
- Trade-offs:
- Higher
fftSize
values provide greater frequency resolution but require more processing power. - Lower values are faster but less detailed.
- Higher
Setting fftSize
to 2048 gives us 1024 meaningful data points for frequency analysis (see below).
AnalyserNode: frequencyBinCount
- The frequencyBinCount is half of the fftSize, representing the number of frequency bins.
- Why only half? FFT: the second half of the data is a mirror image of the first and is, therefore, redundant for analysis.
Using getByteFrequencyData()
- The getByteFrequencyData() method outputs an array where each value represents a specific frequency range's intensity (volume).
- Values range from 0 (silent) to 255 (very loud).
- Mapping Frequency to Array Indices:
- The lower indices represent low frequencies (bass).
- The higher indices represent high frequencies (treble).
Example: Extracting Frequencies for Human Voice
Human speech typically ranges from 80 Hz to 255 Hz, and to isolate this range in the frequency data, we need to map it to specific indices in the getByteFrequencyData() array. Let’s break it down and explain why specific values are calculated as they are:
Step 1: Define Key Parameters
To start, let’s assume the following typical audio settings:
- samplingRate = 44100 Hz: This is the number of audio samples captured per second, the standard for most audio recording applications.
- fftSize = 2048: The Fast Fourier Transform size determines the "resolution" of the frequency analysis. Larger values provide more detailed data but require more processing time. 2048 is the default value.
- frequencyBinCount = fftSize / 2: This gives us the number of frequency bins.
Why is frequencyBinCount half of fftSize?
The FFT produces a set of complex numbers that represent the frequencies in the signal, but the second half of the output is a mirror image of the first half due to the symmetry of the Fourier Transform. This means only the first half contains useful data for frequency analysis, so we divide fftSize
by 2 to get the number of meaningful bins.
For example, if fftSize
is 2048, frequencyBinCount
will be:
frequencyBinCount = fftSize / 2 = 2048 / 2 = 1024
Step 2: Calculate Frequency Per Bin
Each frequency bin in the data array corresponds to a specific frequency range, which is determined by the samplingRate and the number of bins (frequencyBinCount). The formula to calculate the frequency represented by each bin is:
frequencyPerBin = samplingRate / 2 * frequencyBinCount
Why divide the samplingRate by 2?
The Nyquist Theorem tells us that the maximum frequency we can measure is half the sampling rate (called the Nyquist frequency). Dividing the samplingRate by 2 gives the range of frequencies from 0 Hz to the Nyquist frequency, which we want to analyze.
Substituting frequencyBinCount
= 1024 and samplingRate
= 44100 Hz:
frequencyPerBin = 44100 / 2 * 1024 = 44100 / 2048 ≈ 21.53 Hz per bin
This tells us that each index in the array corresponds to a frequency range of approximately 21.53 Hz. For example:
- Index 0 represents frequencies from 0 to 21.53 Hz.
- Index 1 represents frequencies from 21.53 Hz to 43.06 Hz, and so on.
Step 3: Determine Index Range for Human Voice
Now that we know the frequency represented by each bin, we can calculate the indices corresponding to the range of human conversation (80 Hz to 255 Hz).
Start Index (80 Hz):
startIndex = startFrequency / frequencyPerBin = 80 / 21.53 ≈ 4
End Index (255 Hz):
endIndex = endFrequency / frequencyPerBin = 255 / 21.53 ≈ 12
This means that the relevant portion of the array for human speech frequencies lies between indices 4 and 12. By extracting this subset of the data, we can focus on analyzing the loudness or presence of frequencies in the range typically used for human speech.
Why Does This Matter?
By understanding how to map frequencies to array indices, we can tailor audio processing tasks like:
- Voice Isolation: Extracting human voice frequencies for conferencing or transcription.
- Frequency Filtering: Ignoring unwanted noise outside the range of interest.
- Audio Visualization: Creating meaningful visualizations that emphasize specific ranges of sound.
Practical Implementation
A practical implementation can be found in this CodePen demo.
Conclusion: Why Use Frequency Analysis?
The AnalyserNode and getByteFrequencyData() provide developers with powerful tools to dive deep into audio processing, and with a good implementation, these tools can improve any web-based audio application.