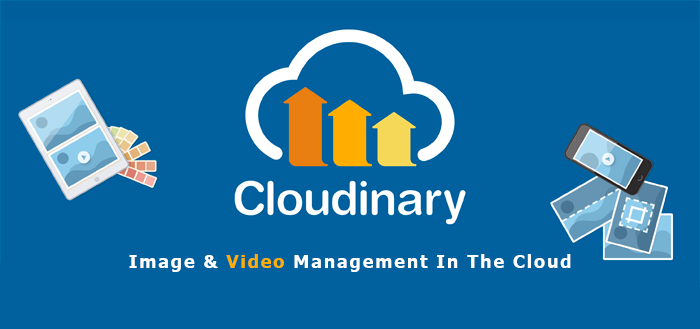
Smarter Video Hosting & Delivery With Cloudinary
When it comes to storing your (newly recorded) videos Amazon’s S3 is the obvious choice and it’s a great choice. But Cloudinary’s video platform is a lot more powerful when it comes to delivering those videos to your users, across the world, on thousands of different devices, including powerful new Macs, low-powered mobile devices connected over 3G, TVs and desktop computers running older browsers.
What makes Cloudinary different?
Two things that set Cloudinary apart are the abilities to:
- scale, crop, trim, rotate, convert to and from animated GIF, and transcode the original video dynamically on-the-fly
- control the above video transformations directly from the playback URL
These features give you immediate flexibility to:
- deliver the right video format to each browser and device
- ensure smooth playback without interruptions, no matter the bandwidth,
- make sure that the video is not only decodable, but also playable.
Pushing videos to Cloudinary’s servers
The Pipe video recording platform does not integrate directly (yet) but Cloudinary provides a secure API for easily uploading videos from:
- server-side code
- directly from the browser
- a mobile app
Uploading from server side using PHP is as simple as using the code below
<?php
require 'Cloudinary.php';
require 'Uploader.php';
require 'Api.php';
Cloudinary::config(array(
"cloud_name" => "CLOUD-NAME",
"api_key" => "API-KEY",
"api_secret" => "API-SECRET"
));
$result = CloudinaryUploader::upload("duck.mp4", array(
"resource_type" => "video"
));
The PHP SDK files can be found here and Cloudinary’s documentation has examples for Ruby, Node.js, Java, Python & .Net.
The code above will upload the duck.mp4 file to Cloudinary’s servers and return a PHP Array containing, among other data, the public HTTPS URL:
The $result
Array above will contain a lot of metadata about the newly uploaded file, including:
- the public HTTP and HTTPS URLs to the newly uploaded file
- the video’s dimensions and duration
- the video format and file size in bytes
- a signature for verifying the response
- various technical audio and video parameters such as codec and bit-rate
This gist contains an example of a full response (PHP Array).
For pushing video files larger than 100MB to Cloudinary make sure you use chunked video uploads.
Modifying a video on-the-fly using the video’s URL
Cloudinary’s dynamic delivery URLs allow you to modify your videos in real-time when accessed by your visitors (lazy transformations).
All transformed videos are generated on-the-fly, stored persistently, cached and delivered through a fast CDN.
Scaling down a video
The duck.mp4 video I uploaded above is a huge 720p video that I want to scale down for use in a mobile website. I’m going to scale it down to 320px in width from 1280px using the c_scale,w_320
query string in the URL.
<video class="wp-video-shortcode" controls="controls" height="180" id="video-1762-7" preload="metadata" width="320">
<source src="https://res.cloudinary.com/addpipe-com/video/upload/c_scale,w_320/v1506088216/zhalp7ahtze0x7gj85sa.mp4?_=7" type="video/mp4"></source>
</video>
Cropping a video
Cropping a video to a 4:3 ratio is as easy as specifying c_crop
in the query string and the desired width and height (320×240 in our case).
<video class="wp-video-shortcode" controls="controls" height="240" id="video-1762-8" preload="metadata" width="320">
<source src="http://res.cloudinary.com/addpipe-com/video/upload/c_crop,h_240,w_320/v1506089598/buijtildd3zzgfg4qozw.mp4?_=8" type="video/mp4"></source>
</video>
Rotating a video
Rotating to a specific angle is possible through the a_XX
query string. Below we’ve both scaled it down and rotated it 90 degrees using a_90
.
<video class="wp-video-shortcode" controls="controls" height="320" id="video-1762-9" preload="metadata" width="180">
<source src="http://res.cloudinary.com/addpipe-com/video/upload/c_scale,w_320/a_90/v1506089598/buijtildd3zzgfg4qozw.mp4?_=9" type="video/mp4"></source>
</video>
Converting to GIF
Converting a video to a GIF file is as simple as ending URL with .gif.
You can combine the conversion to GIF with a trim operation (through the start offset and end offset query string options) to create a smaller gif with only the section of the video that you’re interested. In the below example I am trimming the first 10 seconds (from offset 0 to offset 10).
Transcoding to MP4
If your video is an old .avi or .wmv file, you can easily transcode it on-the-fly to an MP4 file containing H.264 video and AAC audio that’s playable on most modern browsers using the .mp4 ending and the ac_aac
+ vc_h264
query strings in the URL.
Other video transformations
Cloudinary’s solution also enables you to add text captions and subtitles, extract a snapshot, add watermarks or image and video overlays, concatenate or trim videos, stream through HLS and MPEG-DASH and a lot more. For the complete list check out their documentation on video manipulation and delivery.
Eliminating conversion time
Since all Cloudinary video transformations are applied on-the-fly it takes time to deliver a transformed video from the moment it’s requested through the URL. The time required for generating a transformed video depends on various factors, such as original video dimensions, file size, requested dimensions and applied effects, and it can take up to several seconds or even longer.
But if you already know the transformations you need to apply to a video Cloudinary can help by applying them when the video is initially uploaded.
Modifying a video during upload
Cloudinary can modify the video during the video upload process by using eager transformations. The eager approach requires a little planning, but is highly recommended if you have to make sure that all transformed videos are ready for fast delivery even for the first visitors to your videos.
Cloudinary can apply these eager video transformations:
- during the upload process (for files smaller than 40MB),
- or asynchronously after the video has been uploaded.
The modified video can be saved as a new video along the original one or it can be stored as the original video (incoming transformations).
Video CDN
By default Cloudinary uses the Akamai CDN for quick delivery from a widespread network of edge servers around the world. This means with videos hosted with Cloudinary will load faster regardless of the viewer’s location.
With S3 the videos are physically located in the chosen region for the S3 bucket. The farther a user is from that specific region the slower the videos will load. For faster load times S3 buckets can be linked to CloudFront, Amazon’s CDN solution.
Integrating Pipe With Cloudinary
Pipe does not (yet) integrate directly with Cloudinary but one could easily set up a server side script to push the newly recorded video to Cloudinary’s servers. The script can be triggered by one of Pipe’s storage webhook events like video_copied_ftp
or video_copied_s3
which fire when the video is stored on your web server or S3 bucket respectively and thus available to be pushed forward to Cloudinary for transformations and delivery.